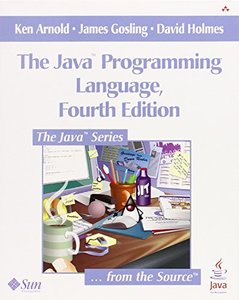
The Java Programming Language, 4/e (Paperback)
內容描述
Table of
Contents:
Preface xxi
Chapter 1: A Quick Tour
1
1.1 Getting Started
11.2 Variables 31.3 Comments in
Code 61.4 Named Constants
71.5 Unicode Characters 81.6 Flow of
Control 91.7 Classes and Objects
121.8 Methods and Parameters
151.9 Arrays 181.10 String
Objects 211.11 Extending a Class
241.12 Interfaces 271.13 Generic
Types 291.14 Exceptions
321.15 Annotations
351.16 Packages 361.17 The Java
Platform 381.18 Other Topics Briefly
Noted 39
Chapter 2: Classes and Objects
41
2.1 A Simple Class
422.2 Fields 442.3 Access
Control 472.4 Creating Objects
492.5 Construction and Initialization
502.6 Methods
562.7 this
682.8 Overloading Methods
692.9 Importing Static Member Names
712.10 The main Method
732.11 Native Methods 74
Chapter 3: Extending Classes
75
3.1 An Extended Class
763.2 Constructors in Extended Classes
803.3 Inheriting and Redefining Members
843.4 Type Compatibility and Conversion
903.5 What protected Really Means
933.6 Marking Methods and Classes
final 963.7 Abstract Classes and
Methods 973.8 The Object
Class 993.9 Cloning Objects
1013.10 Extending Classes: How and When
1073.11 Designing a Class to Be Extended
1083.12 Single Inheritance versus Multiple
Inheritance 114
Chapter 4: Interfaces
117
4.1 A Simple Interface
Example 1184.2 Interface Declarations
1204.3 Extending Interfaces
1224.4 Working with Interfaces
1264.5 Marker Interfaces 1304.6 When
to Use Interfaces 131
Chapter 5: Nested Classes and
Interfaces 133
5.1 Static Nested Types
1335.2 Inner Classes 1365.3 Local
Inner Classes 1425.4 Anonymous Inner
Classes 1445.5 Inheriting Nested Types
1465.6 Nesting in Interfaces
1485.7 Implementation of Nested Types
149
Chapter 6: Enumeration Types
151
6.1 A Simple Enum Example
1516.2 Enum Declarations 1526.3 Enum
Constant Declarations 1546.4 java.lang.Enum
1596.5 To Enum or Not
160
Chapter 7: Tokens, Values, and
Variables 161
7.1 Lexical Elements
1617.2 Types and Literals
1667.3 Variables 1697.4 Array
Variables 1737.5 The Meanings of Names
178
Chapter 8: Primitives as Types
183
8.1 Common Fields and
Methods 1848.2 Void
1878.3 Boolean
1878.4 Number
1888.5 Character
1928.6 Boxing Conversions 198
Chapter 9: Operators and Expressions
201
9.1 Arithmetic Operations
2019.2 General Operators
2049.3 Expressions 2149.4 Type
Conversions 2169.5 Operator Precedence and
Associativity 2219.6 Member Access
223
Chapter 10: Control Flow 229
10.1 Statements and Blocks
22910.2 if-else
23010.3 switch
23210.4 while and do-while
23510.5 for
23610.6 Labels 24110.7 break
24110.8 continue
24410.9 return
24510.10 What, No goto? 246
Chapter 11: Generic Types
247
11.1 Generic Type
Declarations 25011.2 Working with Generic
Types 25611.3 Generic Methods and
Constructors 26011.4 Wildcard Capture
26411.5 Under the Hood: Erasure and Raw Types
26711.6 Finding the Right Method--Revisited
27211.7 Class Extension and Generic Types
276
Chapter 12: Exceptions and Assertions
279
12.1 Creating Exception
Types 28012.2 throw
28212.3 The throws Clause
28312.4 try, catch, and
finally 28612.5 Exception
Chaining 29112.6 Stack Traces
29412.7 When to Use Exceptions
29412.8 Assertions 29612.9 When to
Use Assertions 29712.10 Turning Assertions On and
Off 300
Chapter 13: Strings and Regular
Expressions 305
13.1 Character Sequences
30513.2 The String Class
30613.3 Regular Expression Matching
32113.4 The StringBuilder Class
33013.5 Working with UTF-16 336
Chapter 14: Threads 337
14.1 Creating Threads
33914.2 Using Runnable
34114.3 Synchronization
34514.4 wait, notifyAll, and
notify 35414.5 Details of Waiting and
Notification 35714.6 Thread Scheduling
35814.7 Deadlocks 36214.8 Ending
Thread Execution 36514.9 Ending Application
Execution 36914.10 The Memory Model:
Synchronization and volatile
37014.11 Thread Management, Security, and ThreadGroup
37514.12 Threads and Exceptions
37914.13 ThreadLocal Variables
38214.14 Debugging Threads 384
Chapter 15: Annotations
387
15.1 A Simple Annotation
Example 38815.2 Annotation Types
38915.3 Annotating Elements
39215.4 Restricting Annotation Applicability
39315.5 Retention Policies
39515.6 Working with Annotations 395
Chapter 16: Reflection
397
16.1 The Class
Class 39916.2 Annotation Queries
41416.3 The Modifier Class
41616.4 The Member classes
41616.5 Access Checking and
AccessibleObject 41716.6 The
Field Class 41816.7 The
Method Class 42016.8 Creating New
Objects and the Constructor Class
42316.9 Generic Type Inspection
42616.10 Arrays
42916.11 Packages 43216.12 The
Proxy Class 43216.13 Loading
Classes 43516.14 Controlling Assertions at
Runtime 444
Chapter 17: Garbage Collection and
Memory 447
17.1 Garbage Collection
44717.2 A Simple Model
44817.3 Finalization
44917.4 Interacting with the Garbage Collector
45217.5 Reachability States and Reference Objects
454
Chapter 18: Packages 467
18.1 Package Naming
46818.2 Type Imports 46918.3 Package
Access 47118.4 Package Contents
47518.5 Package Annotations
47618.6 Package Objects and Specifications
477
Chapter 19: Documentation Comments
481
19.1 The Anatomy of a Doc
Comment 48219.2 Tags
48319.3 Inheriting Method Documentation Comments
48919.4 A Simple Example
49119.5 External Conventions
49619.6 Notes on Usage 497
Chapter 20: The I/O Package
499
20.1 Streams Overview
50020.2 Byte Streams
50120.3 Character Streams
50720.4 InputStreamReader and
OutputStreamWriter 51220.5 A Quick
Tour of the Stream Classes 51420.6 The Data Byte
Streams 53720.7 Working with Files
54020.8 Object Serialization
54920.9 The IOException Classes
56320.10 A Taste of New I/O 565
Chapter 21: Collections
567
21.1 Collections
56721.2 Iteration 57121.3 Ordering
with Comparable and Comparator
57421.4 The Collection Interface
57521.5 Set and SortedSet
57721.6 List
58021.7 Queue
58521.8 Map and SortedMap
58721.9 enum Collections
59421.10 Wrapped Collections and the Collections
Class 59721.11 Synchronized Wrappers and Concurrent
Collections 60221.12 The Arrays
Utility Class 60721.13 Writing Iterator
Implementations 60921.14 Writing Collection
Implementations 61121.15 The Legacy Collection
Types 61621.16 Properties
620
Chapter 22: Miscellaneous Utilities
623
22.1 Formatter
62422.2 BitSet
63222.3 Observer/Observable
63522.4 Random
63922.5 Scanner
64122.6 StringTokenizer
65122.7 Timer and
TimerTask
65322.8 UUID 65622.9
Math and StrictMath 657
Chapter 23: System Programming
661
23.1 The System
Class 66223.2 Creating Processes
66623.3 Shutdown 67223.4 The Rest of
Runtime 67523.5 Security
677
Chapter 24: Internationalization and
Localization 685
24.1 Locale
68624.2 Resource Bundles
68824.3 Currency 69424.4 Time,
Dates, and Calendars 69524.5 Formatting and Parsing
Dates and Times 70324.6 Internationalization and
Localization for Text 708
Chapter 25: Standard Packages 715
25.1 java.awt--The
Abstract Window Toolkit
71725.2 java.applet--Applets
72025.3 java.beans--Components
72125.4 java.math--Mathematics
72225.5 java.net--The Network
72425.6 java.rmi--Remote Method
Invocation 72725.7 java.security and
Related Packages--Security Tools
73225.8 java.sql--Relational Database
Access 73225.9 Utility Subpackages
73325.10 javax.* --Standard Extensions
73725.11 javax.accessibility--Accessibility for
GUIs 73725.12 javax.naming--Directory
and Naming Services
73825.13 javax.sound--Sound
Manipulation
73925.14 javax.swing--Swing GUI
Components
74025.15 org.omg.CORBA--CORBA APIs
740
Appendix A: Application Evolution
741
A.1 Language, Library, and Virtual
Machine Versions 741A.2 Dealing with Multiple
Dialects 743A.3 Generics: Reification, Erasure, and
Raw Types 744
Appendix B: Useful Tables
749
Further Reading
755
Index
761